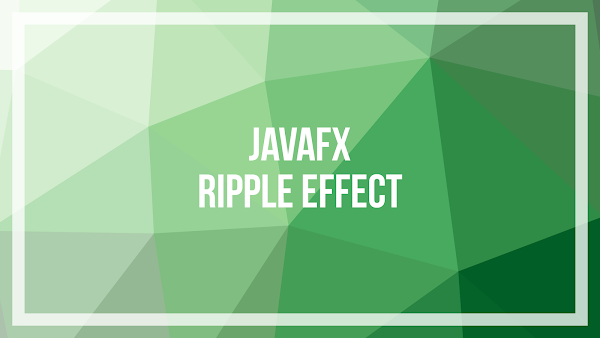
import javafx.animation.ScaleTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ContentDisplay;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import javafx.util.Duration;
/**
* JavaFX App
*/
public class App extends Application {
@Override
public void start(Stage stage) {
// Bouton avec l'effet
Button btn = new Button("Ripple effect");
// Bouton bleu et texte blanc
btn.setStyle("-fx-background-color: #007bff; -fx-text-fill: white; -fx-font-weight: bold;");
// Cercle qui va s'animer
Circle circle = new Circle(0);
// Le cercle ne doit pas dépasser la taille du bouton
Rectangle clip = new Rectangle();
clip.widthProperty().bind(btn.widthProperty());
clip.heightProperty().bind(btn.heightProperty());
btn.setClip(clip);
// Cercle blanc transparent
circle.setFill(Color.color(1, 1, 1, 0.2));
// Animation
ScaleTransition scaleTransition = new ScaleTransition(Duration.millis(250), circle);
// Le cercle s'agrandit comme la largeur et hauteur du bouton
scaleTransition.toXProperty().bind(btn.widthProperty());
scaleTransition.toYProperty().bind(btn.heightProperty());
// Animation aller / retour
scaleTransition.setAutoReverse(true);
scaleTransition.setCycleCount(2);
// Le cercle au centre du bouton
btn.setGraphic(circle);
btn.setContentDisplay(ContentDisplay.CENTER);
// Lors d'un clic sur le bouton
btn.setOnAction(event -> {
circle.setRadius(1);
scaleTransition.play();
});
Scene scene = new Scene(new StackPane(btn), 640, 480);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch();
}
}
Commentaires
Enregistrer un commentaire